Particionamiento con LeavePOut#
Ultima modificación: 2023-02-27 | `YouTube <>`__
[1]:
from sklearn.model_selection import LeavePOut
leavePOut = LeavePOut(
# --------------------------------------------------------------------------
# Tamaño del conjunto de salida
p=3,
)
leavePOut
[1]:
LeavePOut(p=3)
[2]:
from mymodule import plot_schema
y_classes = [0] * 10 + [1] * 10
plot_schema(leavePOut, y_classes)
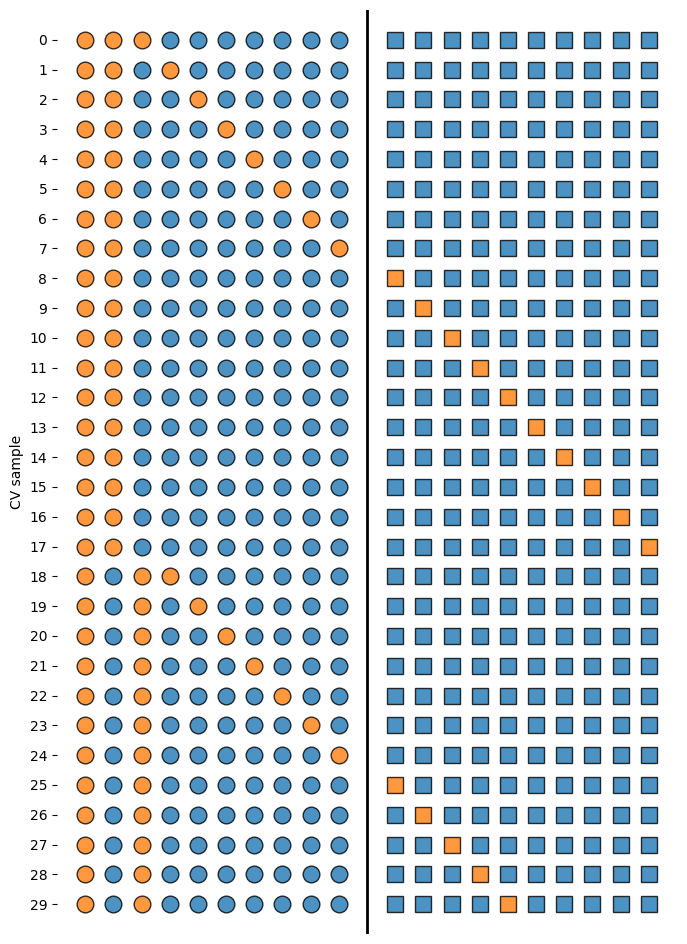
[3]:
leavePOut.get_n_splits(y_classes)
[3]:
1140
[4]:
import numpy as np
X = np.array([[1, 2], [3, 4], [5, 6], [7, 8], [9, 10], [11, 12]])
y = np.array([1, 2, 3, 4, 5, 6])
leavePOut = LeavePOut(p=2)
for i, (train_index, test_index) in enumerate(leavePOut.split(X)):
print(f"Fold {i}:")
print(f" Train: index={train_index}")
print(f" Test: index={test_index}")
print()
Fold 0:
Train: index=[2 3 4 5]
Test: index=[0 1]
Fold 1:
Train: index=[1 3 4 5]
Test: index=[0 2]
Fold 2:
Train: index=[1 2 4 5]
Test: index=[0 3]
Fold 3:
Train: index=[1 2 3 5]
Test: index=[0 4]
Fold 4:
Train: index=[1 2 3 4]
Test: index=[0 5]
Fold 5:
Train: index=[0 3 4 5]
Test: index=[1 2]
Fold 6:
Train: index=[0 2 4 5]
Test: index=[1 3]
Fold 7:
Train: index=[0 2 3 5]
Test: index=[1 4]
Fold 8:
Train: index=[0 2 3 4]
Test: index=[1 5]
Fold 9:
Train: index=[0 1 4 5]
Test: index=[2 3]
Fold 10:
Train: index=[0 1 3 5]
Test: index=[2 4]
Fold 11:
Train: index=[0 1 3 4]
Test: index=[2 5]
Fold 12:
Train: index=[0 1 2 5]
Test: index=[3 4]
Fold 13:
Train: index=[0 1 2 4]
Test: index=[3 5]
Fold 14:
Train: index=[0 1 2 3]
Test: index=[4 5]