El dataset hand-written digits — 2:48 min#
2:48 min | Ultima modificación: Septiembre 28, 2021 | YouTube
https://scikit-learn.org/stable/datasets/toy_dataset.html
https://archive.ics.uci.edu/ml/datasets/Optical+Recognition+of+Handwritten+Digits.html
Este dataset contiene las imágenes procesadas de dígitos escrítos a mano por un grupo de 43 personas. Los datos de entrenamiento provienen de 30 personas, mientras que los de prueba provienen de las 13 restantes.
Las imágenes fueron extraídas de bitmaps de 32 bits. Estos bitmaps fueron divididos en bloques no traslapados de 4x4 pixels. Para cada bloque se reporta la cantidad de pixels (entero entre 0 y 16), obteniendose así una muestra de 8x8.
El dataset contiene 1797 instancias, con aproximadamente 180 muestras para cada dígito.
[1]:
from sklearn.datasets import load_digits
[2]:
bunch = load_digits(
# -------------------------------------------------------------------------
# The number of classes to return. Between 0 and 10.
n_class=10,
# -------------------------------------------------------------------------
# If True, returns (data, target) instead of a Bunch
# object.
return_X_y=False,
)
bunch.keys()
[2]:
dict_keys(['data', 'target', 'target_names', 'images', 'DESCR'])
[3]:
bunch.data.shape
[3]:
(1797, 64)
[4]:
import matplotlib.pyplot as plt
fig, axs = plt.subplots(nrows=10, ncols=10, figsize=(6, 6))
for idx, ax in enumerate(axs.ravel()):
ax.imshow(bunch.data[idx].reshape((8, 8)), cmap=plt.cm.binary)
ax.axis("off")
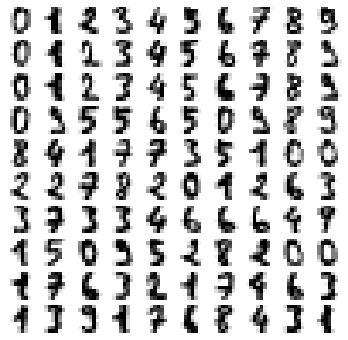
[5]:
bunch.data[0]
[5]:
array([ 0., 0., 5., 13., 9., 1., 0., 0., 0., 0., 13., 15., 10.,
15., 5., 0., 0., 3., 15., 2., 0., 11., 8., 0., 0., 4.,
12., 0., 0., 8., 8., 0., 0., 5., 8., 0., 0., 9., 8.,
0., 0., 4., 11., 0., 1., 12., 7., 0., 0., 2., 14., 5.,
10., 12., 0., 0., 0., 0., 6., 13., 10., 0., 0., 0.])
[6]:
bunch.target
[6]:
array([0, 1, 2, ..., 8, 9, 8])
[7]:
bunch.target_names
[7]:
array([0, 1, 2, 3, 4, 5, 6, 7, 8, 9])
[8]:
X, y = load_digits(
# -----------------------------------------------------
# The number of classes to return. Between 0 and 10.
n_class=10,
# -----------------------------------------------------
# If True, returns (data, target) instead of a Bunch
# object.
return_X_y=True,
)
display(
X[:5, :],
y[:5],
)
array([[ 0., 0., 5., 13., 9., 1., 0., 0., 0., 0., 13., 15., 10.,
15., 5., 0., 0., 3., 15., 2., 0., 11., 8., 0., 0., 4.,
12., 0., 0., 8., 8., 0., 0., 5., 8., 0., 0., 9., 8.,
0., 0., 4., 11., 0., 1., 12., 7., 0., 0., 2., 14., 5.,
10., 12., 0., 0., 0., 0., 6., 13., 10., 0., 0., 0.],
[ 0., 0., 0., 12., 13., 5., 0., 0., 0., 0., 0., 11., 16.,
9., 0., 0., 0., 0., 3., 15., 16., 6., 0., 0., 0., 7.,
15., 16., 16., 2., 0., 0., 0., 0., 1., 16., 16., 3., 0.,
0., 0., 0., 1., 16., 16., 6., 0., 0., 0., 0., 1., 16.,
16., 6., 0., 0., 0., 0., 0., 11., 16., 10., 0., 0.],
[ 0., 0., 0., 4., 15., 12., 0., 0., 0., 0., 3., 16., 15.,
14., 0., 0., 0., 0., 8., 13., 8., 16., 0., 0., 0., 0.,
1., 6., 15., 11., 0., 0., 0., 1., 8., 13., 15., 1., 0.,
0., 0., 9., 16., 16., 5., 0., 0., 0., 0., 3., 13., 16.,
16., 11., 5., 0., 0., 0., 0., 3., 11., 16., 9., 0.],
[ 0., 0., 7., 15., 13., 1., 0., 0., 0., 8., 13., 6., 15.,
4., 0., 0., 0., 2., 1., 13., 13., 0., 0., 0., 0., 0.,
2., 15., 11., 1., 0., 0., 0., 0., 0., 1., 12., 12., 1.,
0., 0., 0., 0., 0., 1., 10., 8., 0., 0., 0., 8., 4.,
5., 14., 9., 0., 0., 0., 7., 13., 13., 9., 0., 0.],
[ 0., 0., 0., 1., 11., 0., 0., 0., 0., 0., 0., 7., 8.,
0., 0., 0., 0., 0., 1., 13., 6., 2., 2., 0., 0., 0.,
7., 15., 0., 9., 8., 0., 0., 5., 16., 10., 0., 16., 6.,
0., 0., 4., 15., 16., 13., 16., 1., 0., 0., 0., 0., 3.,
15., 10., 0., 0., 0., 0., 0., 2., 16., 4., 0., 0.]])
array([0, 1, 2, 3, 4])